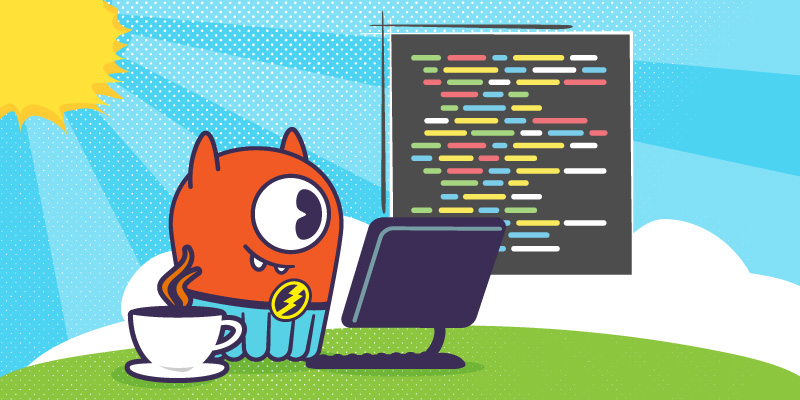
IMPORTANT: Since the first publication of the Mutant Monitoring System we have made a number of updates to the concepts and code presented in the blog series. You can find the latest version now in ScyllaDB University. Click here to be directed to the new version. |
This is part 11 of a series of blog posts that provides a story arc for ScyllaDB Training.
In the previous post, we explained how a ScyllaDB Administrator can backup and restore a cluster. As the number of mutants is on the rise, Division 3 decided that we must use more applications to connect to the mutant catalog and decided to hire Java developers to create powerful applications that can monitor the mutants. In this post, we will explore how to connect to a ScyllaDB cluster using the Cassandra driver for Java.
When creating applications that communicate with a database such as ScyllaDB, it is crucial that the programming language being used has support for database connectivity. Since ScyllaDB is compatible with Cassandra, we can use any of the available Cassandra libraries. For example in Go, there is Gocql and Gocqlx. In Node.js, there is cassandra-driver. For Java, we have the driver available from Datastax. Since Division 3 wants to start investing in Java, let’s begin by writing a sample Java application.
Creating a Sample Java Application
The sample application that we will go over will connect to a ScyllaDB cluster, display the contents of the Mutant Catalog table, insert and delete data, and show the contents of the table after each action. We will first go through each section of the code and then explain how to run the code in a Docker container that will access the ScyllaDB Mutant Monitoring cluster.
In the application, we first need to import the Datastax Cassandra driver for Java:
The Cluster library provides the ability to connect to the cluster. The ResultSet library will allow us to run queries such as SELECT statements and store the output. The Row library allows us to get the value of a CQL Row returned in a ResultSet. The session library provides the ability to connect to a specific keyspace.
For this application, the main class is called App and the code should be stored in a file called App.java. After the class is defined, we can begin defining which cluster and keyspace to use:
To display the data, we will need to create a function that will run a select statement from the ScyllaDB cluster. The function below will use the ResultSet, Session, and Row libraries to gather the data and print it on the screen:
The next function is used to insert a new Mutant into the Mutant Catalog table. Since this is a simple query, only the Session library is used. After the insert takes place, the previous function, selectQuery()
, is called to display the contents of the table after the new data is added.
After the data is added and displayed in the terminal, we will delete it and then display the contents of the table again:
This is the main method of the application and is the starting point for any Java application. In this function, we will add the functions that were created above to be executed in the order below. When all of the functions are completed, the application will exit.
With the coding part done, let’s bring up the ScyllaDB Cluster and then run the sample application in Docker.
Starting the ScyllaDB Cluster
The ScyllaDB Cluster should be up and running with the data imported from the previous blog posts.
The MMS Git repository has been updated to provide the ability to automatically import the keyspaces and data. If you have the Git repository already cloned, you can simply do a “git pull” in the scylla-code-samples directory.
git clone https://github.com/scylladb/scylla-code-samples.git
cd scylla-code-samples/mms
Modify docker-compose.yml and add the following line under the environment: section of scylla-node1:
- IMPORT=IMPORT
Now we can build and run the containers:
docker-compose build
docker-compose up -d
After roughly 60 seconds, the existing MMS data will be automatically imported. When the cluster is up and running, we can run our application code.
Building and Running the Java Example
To build the application in Docker, change into the java subdirectory in scylla-code-samples:
cd scylla-code-samples/mms/java
Now we can build and run the container:
docker build -t java .
docker run -d --net=mms_web --name java java
To connect to the shell of the container, run the following command:
docker exec -it java sh
Finally, the sample Java application can be run:
java -jar App.jar
The output of the application will be:
Conclusion
In this post, we explained how to create a sample Java application that executes a few basic CQL statements with a ScyllaDB cluster using the Datastax Cassandra driver. This is only the basics and there are more interesting topics that Division 3 wants developers to explore. In the next post, we will go over prepared statements using the Java driver.
In the meantime, please be safe out there and continue to monitor the mutants!
Next Steps
- Learn more about ScyllaDB from our product page.
- See what our users are saying about ScyllaDB.
- Download ScyllaDB. Check out our download page to run ScyllaDB on AWS, install it locally in a Virtual Machine, or run it in Docker.